Create Types
Create Types
Fast and convenient with SDL or Type instances
Edit Types
Edit Types
Generate Types and modify them for better form
Type Registry
Type Registry
All created types avaliable in SchemaComposer storage
Static Analysis
Static Analysis
Includes Flowtype & TypeScript definitions
Amazing Plugins
Amazing Plugins
Plugin may generate and modify your types
Additional Types
Additional Types
Commonly used basic types Date
, JSON
import { schemaComposer } from 'graphql-compose';
const AuthorTC = schemaComposer.createObjectTC({
posts: {
// wrapping Type with arrow function helps to solve a hoisting problem
// also using type instances provides better DX
// (ctrl+click allows to jump to PostTC type declaration in your IDE)
type: () => PostTC,
description: 'Posts written by Author',
resolve: (source, args, context, info) => {},
},
// using standard GraphQL Type
ucFirstName: {
type: GraphQLString,
resolve: (source) => { return source.firstName.toUpperCase(); },
// also request `firstName` field which must be loaded from database
projection: { firstName: true },
},
// fast way if you need to define only type
counter: 'Int',
// using SDL for definition new ObjectType
complex: `type ComplexType {
subField1: String
subField2: Float
subField3: Boolean
subField4: ID
subField5: JSON
subField6: Date
}`,
// SDL for defining array of strings, which is NonNull
list0: {
type: '[String]!',
description: 'Array of strings',
},
list1: '[String]',
list2: ['String'],
list3: [GraphQLString],
list4: [`type Complex2Type { f1: Float, f2: Int }`],
});
- import { makeExecutableSchema } from 'graphql-tools';
+ import { schemaComposer } from 'graphql-compose';
- export const schema = makeExecutableSchema({
- typeDefs,
- resolvers,
- });
+ schemaComposer.addTypeDefs(typeDefs);
+ schemaComposer.addResolveMethods(resolvers);
+ export const schema = schemaComposer.buildSchema();
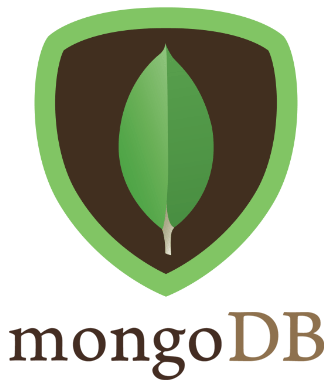
graphql-compose-mongoose
graphql-compose-mongoose
Derives GraphQLType from your mongoose model. Also derives bunch of internal GraphQL Types. Provide convenient CRUD resolvers, including relay connection and pagination.
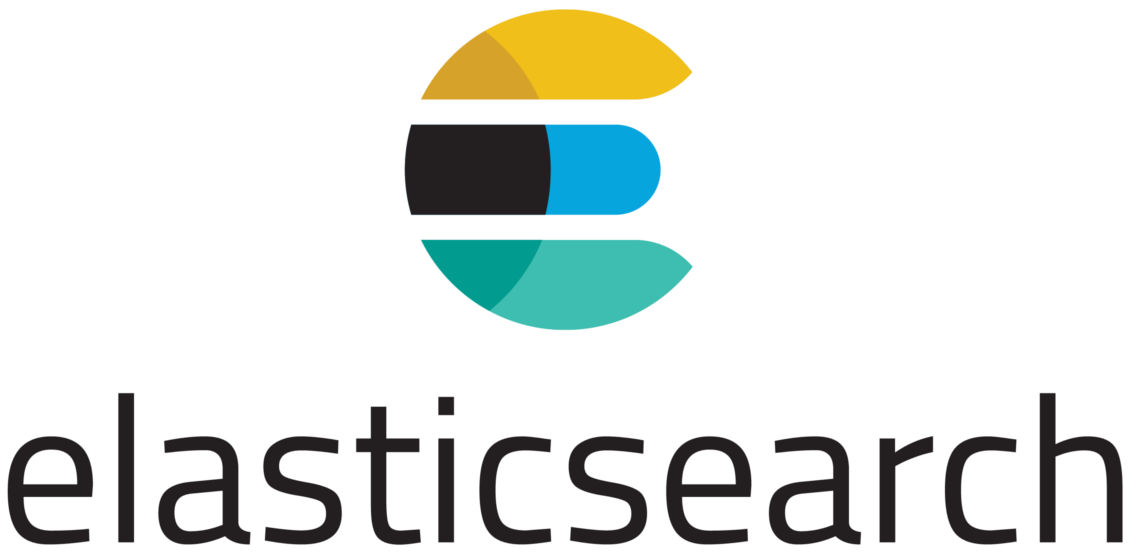
graphql-compose-elasticsearch
graphql-compose-elasticsearch
Derives GraphQLType from your elastic mapping. Generates tons of types, provides all available methods in QueryDSL, Aggregations, Sorting with field autocompletion according to types in your mapping (like Dev Tools Console in Kibana).
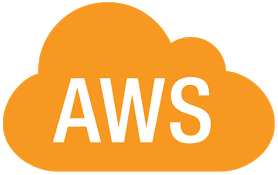
graphql-compose-aws
graphql-compose-aws
Expose AWS Cloud API via GraphQL. Internally it generates Types and FieldConfigs from AWS SDK configs. You may put this generated types to any GraphQL Schema.